A class whose number of instances that can be instantiated is limited to one is called a singleton class. Thus, at any given time only one instance can exist, no more.
The singleton design pattern is used whenever the design requires only one instance of a class. Some examples:
- Application classes. There should only be one application class.
- Logger classes. For logging purposes of an application there is usually one logger instance required.
There are several ways of creating a singleton class. The most simple approach is shown below:
class Singleton
{
public:
static Singleton& Instance()
{
static Singleton singleton;
return singleton;
}
// Other non-static member functions
private:
Singleton() {}; // Private constructor
Singleton(const Singleton&); // Prevent copy-construction
Singleton& operator=(const Singleton&); // Prevent assignment
};
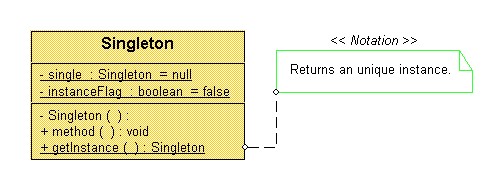
class Singleton{private:static bool instanceFlag;static Singleton *single;Singleton(){//private constructor}public:static Singleton* getInstance();void method();~Singleton(){instanceFlag = false;}};
So the logic behind designing this is it will first check for the instance of the class if the instance is already created it will just return the instance if not present a new single instance will be created.
No comments:
Post a Comment